My first article in the series for beginner programmers was a presentation of the top things to learn when starting to learn programming. This previous article was presenting 3 different tools, and they all will be extremely useful in your everyday life as a programmer, from beginner to professional. However, those are not the only 3 tools I use when programming at work or for my personal projects, and I wanted to go over some of my favorite tools that I use as a professional software engineer.
I will leave Google and Git to the side, because I already talked about them about them in my previous article, but they are of course primordial tools.
Fish shell
If you are just starting to learn programming you might have no had to use it already, but the command line is almost mandatory when developing software. It doesn’t matter which language you use, most will require you to use the terminal some times, to generate some file template, install a new dependency, or just run your application in local.
By default, on Mac and Linux, you will be using the bash shell (the software that runs in the black window where you type your commands), and on Windows you will have access to “cmd”, Powershell, or, with recent releases of Windows 10, a native Linux environment equivalent.
Bash is fine and you can do anything with it, but I prefer an alternative with great features: fish shell.
Fish will increase your productivity dramatically, mainly thanks to one particular feature: the autocompletion of commands. It has smart autocompletion of the commands that you are typing, by looking in your history and in the list of installed programs, just like the address bar in a web-browser.
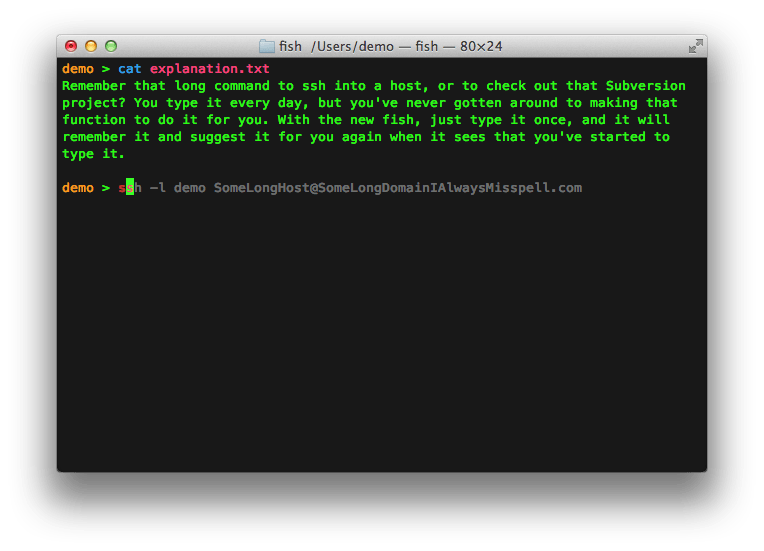
Screen
Screen is also related to using the command line, but this time is a small program that will run in your shell, instead of a shell itself. What you have probably encountered before if you use the command line, is that you are running so many things at the same time, and you need to keep 4 or 5 terminal windows open. Worse, if you are starting your development server from the command line (for Angular, React, Express.js, any Java webserver, etc.), you will have to keep a terminal window open, just to keep that command running. Maybe you’ve even lost work in progress, because a slow command was running and your internet connection dropped after 30 minutes!
What would be great would be to have a tool that allows you to start those commands, let them run, but in the background, without requiring to keep any terminal window open! Screen is that tool. It is a simple command that you install using your favorite package manager on Mac or Linux (brew install screen
for example), and that you can then invoke to run and control terminals running in the background.
Once installed, you only have a few commands to know in order to use it.
$ screen
This will start a new screen session, which is basically a terminal in your terminal. What you will see opening is a new terminal, just like a normal one, where you can run any command.
Once the screen is running, you have a few controls available, that work like keyboard shortcuts instead of typing a command. All of them require you to press Ctrl + A
, release the keys, and then press another key:
Ctrl + A
andC
: This will create a new tab in the screen window. You can have multiple tabs in the same terminal, and they will be shown at the bottom of your terminal window.Ctrl + A
andP
orCtrl + A
andN
: This will navigate to the previous (P) or the next (N) tab available in your screen window.Ctrl + A
andD
: This is the most important, it allows you to close the screen window, without killing the tabs that are open. This is how you keep your programs running even without an active terminal!D
stands for “detach”, which means you are detaching your terminal from that screen window.
There are a few more of those “shortcuts” available, that you can access by typing the command man screen
in your terminal, but I never had to use them.
If you want to reattach your current terminal to a previously open screen that you have closed using Ctrl + A
and D
, you have access to two commands:
$ screen -ls There is a screen on: 17813.ttys003.8c8590ba7933 (Detached) 1 Socket in /var/folders/93/hdmjxc013_9f9xvj_5dbcl0s4f1pk7/T/.screen.
This command, as you can see from the output above, tells you which screens you have available, and wether they are already attached to a terminal window or not.
Then, once you know which screen you want to resume to, use the following command (the ID is optional if you only have one screen instance running):
$ screen -r <id of the screen to reattach>
Chrome Dev tool
If you are building websites, it is primordial to know how to use the Chrome Dev tool (or the Firefox equivalent, which is extremely similar). You might have already used it before to edit the content of some text displayed on a webpage. It is accessed by right-clicking anywhere on a webpage and selecting “Inspect element” in the menu that opens (or by pressing F12).
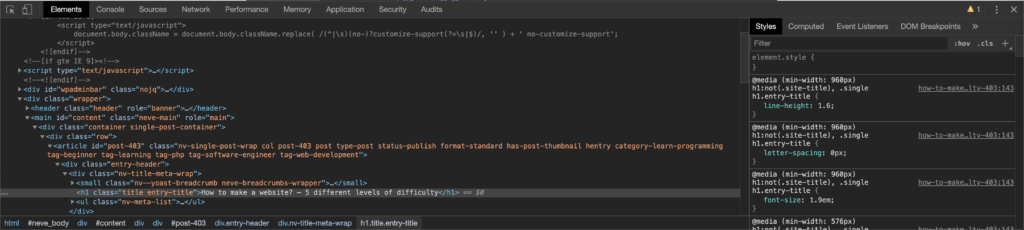
I have seen non-programmers use it before to make a prank by modifying the content of a webpage and taking a screenshot for example, but it is extremely valuable for web developers. I use it all day long when I am working on some frontend task at work, specifically for 3 features:
- The “Elements” tab, which opens when you right click an element on the page and click “Inspect element” will display the current HTML code of the page. If you select an element, you can modify its code, like by adding a CSS class for example. Talking about CSS, the right side of this pane tab allows you to see and modify all the CSS properties of this element. It is incredibly useful when you want to text a color, or some margins on an element.
- The “Console” tab, which is used when developing in JavaScript. It will simply show you all the logs generated by your application, including
console.log()
instructions that you put in your code, but also errors that you have done or that occur for some reason. - The “Network” tab, which displays all the HTTP requests made on the current page. I usually filter this tab to show only the “XHR” requests (asynchronous requests made to your API for example), and I use it to see what my webservice returns in case of errors. You can also re-execute a request that ran previously, for example when testing a form.
Documentations
I will dedicate a full article to that, but there will be a point in your life as a programmer where you won’t need tutorials anymore. Unless it is on a concept that I have no idea about, I never need a tutorial to show me how to build a specific feature or software. What I need however, is the documentation published by the 3rd party libraries that I use.
Reinventing the wheel is always a bad idea in software development, which is the reason why developers are so keen on using libraries: you get the feature you need, fully tested, maintained by someone else, for free. For example, a standard Angular application will use almost 800 third party open-source libraries maintained by the community (which sometimes causes major issues).
This is great, but since you didn’t write the code yourself, you don’t know how to use it. To get you started you might use a tutorial, which is fine to learn libraries, but a tutorial will never present you all the features. This is why maintainers for open-source libraries are releasing documentation, alongside their code.
Java is the language I use the most, and all the documentation usually looks the same for any library made in this language. You can find an example of JavaDoc for the StringUtils class, maintained by the Apache organization. As you can see it will present you all the classes, methods and properties of the library and how to use them.
The documentations might be a bit hard to read at the start, and you might also struggle to find exactly what to search on Google to find the piece of information, but they are a necessary step when it comes to creating software, so get used to using them quickly.
Jetbrains IDEs
I already talked about them quickly in another article, but they are really the absolute best in their category so I wanted to go over it again. You have probably heard about Visual Studio Code, which is a text editor for programming. It is a great tool, but you can (and should) step up your game and use an IDE. It means Integrated Development Environment, which, more explicitly, means that it is a software that brings you all the tools needed to develop in a particular language:
- A text editor, of course, to write your code. Since most IDEs are tailored for a few specific languages they will provide much more powerful features compared to text editors. For example, the autocompletion of the code will be extremely powerful, by analyzing the entirety of your project and being smart enough to determine all the links between each of your classes and functions.
- A very powerful refactoring engine. Refactoring is returning to code you wrote previously to improve it, but in an IDE the refactoring features offered are usually the automatic renaming of a variable or function for example. If you change the name of a variable that you use in 15 different files on a text editor, you will have to manually go and edit those 15 files. With an IDE you will right-click, select “Rename”, and the name will be changed for you everywhere it is used.
- A debugger. Sometimes printing the content of a variable to understand where a bug comes from is not enough, and sometimes it’s even impossible. A debugger allows you to stop the execution of your program anywhere you want to, and visualize the content of the variables.
- A Git client. Instead of using the command line or an external client to manage your git repository, you can usually do it directly through the IDE.
- An integration with most frameworks. If you are developing your program using a framework, the IDE will usually have special support for it, which will alert you if you are doing a mistake, will help you autocomplete your code, etc.
Of course, different IDEs will provide different features, or different implementations of the same feature. My favorite line of IDEs is the one developed by JetBrains. They offer tools for most of the popular programming languages used nowadays, and they are so good that when I switched to using them during my schools years, it really felt like the software knew what I wanted to do. All of them are great tools and provide the same feature, but they are specialized per language as I said earlier. Here are a few mentions:
- IntelliJ IDEA. It is one of the free IDEs offered by Jetbrains, and it is mainly made for the languages that run on the JVM (Java Virtual Machine), including Java, Kotlin, Groovy and more.
- WebStorm. As the name indicates, it is made for web development, and I use it whenever I have a frontend project, for example using Angular or React. Unfortunately it is not free, but IntelliJ will work for JavaScript.
- PyCharm. This one is the Python version of the IDE, and like IntelliJ for Java, it has a free “community” version.
- DataGrip. This last one is different from the others because it is not used to develop software, but instead to manage databases and write SQL scripts. It allows you to connect to your database, modify them and run queries on them. Just like the other tools it is great, but it isn’t available for free, so I recommend you the free alternative DBeaver, it is a bit more clunky, but it is definitely a commercial-grade software that will do a great job.
There are some other IDEs available for other languages but either I have no experience with them, or they have a more popular alternative, like Visual Studio (not Code) for C++ and C#. I will also quickly mention Eclipse, which is a free IDE and an alternative to all the IDEs I mentioned here if you prefer it (you won’t).
To close this section, I just want to say, Students, rejoice, because JetBrains is giving away for free all of their IDEs to students during the whole course of their studies. Head to the Educational Licenses page and signup with your University’s email to get the free access (it worked with my French school, so most likely it’ll work with any).
And more…
I use quite often some other tools that I either covered in a dedicated article, or are too anecdotical to have a full section, but here’s a list of some of the other web application and software that I like to use in my day to day job:
- PlantUML, a tool to make UML diagrams easily (dedicated article). UMLs are diagrams that represent your application before you start coding: the use-cases of the users, how each component will work together, etc.
- Regex101, an online regex checker and explainer. If you are not familiar, regex are a way to match a text that you submit with some rules that the developer creates. For example, you can check that the user submitted the word “MindFlash” in a text that he wrote. Regex101 is a tool that allows you to write your regex online and it will automatically explain you each part, which i very useful when writing complex regex.
- Trello, a web application to manage your tasks. It is extremely famous and you can use it to manage any project, even non-programming related ones. It allows you to create cards that represent your tasks that you can then categorize in order to track their status.
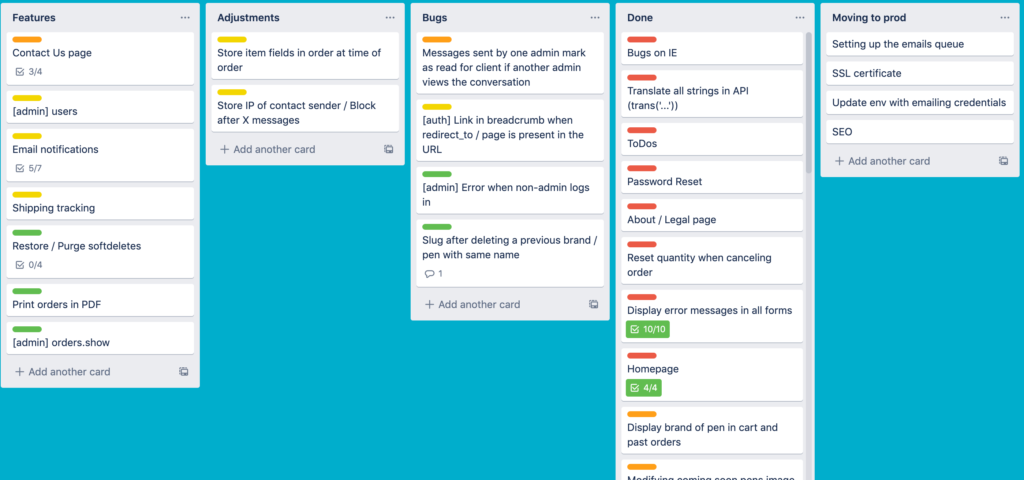
To conclude, I would like to say that my motivation behind writing this article is not only to present some great tools that you can leverage, but also to show you that there is no “secret software” that professional developers use and that makes them get programming super power. All of those tools will make you more efficient or make your time spent developing more pleasant, but the only thing that will make you become a good developer is practice. There is no secret formula, and no shortcut.